Classification Example
[1]:
import os
import sys
from base64 import b64encode
import pathlib
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
from functools import partial
import albumentations as albu
Matplotlib created a temporary config/cache directory at /tmp/matplotlib-14mjotfm because the default path (/.config/matplotlib) is not a writable directory; it is highly recommended to set the MPLCONFIGDIR environment variable to a writable directory, in particular to speed up the import of Matplotlib and to better support multiprocessing.
Require 24 GB of GPU RAM
[2]:
gpus = tf.config.experimental.list_physical_devices("GPU")
#os.environ['TF_GPU_ALLOCATOR'] = 'cuda_malloc_async'
if gpus:
try:
tf.config.experimental.set_virtual_device_configuration(
gpus[0],
[
tf.config.experimental.VirtualDeviceConfiguration(
memory_limit=1024 * 24
)
],
)
except RuntimeError as e:
print(e)
Download and setup dataset
[3]:
flowers_root = tf.keras.utils.get_file(
'flower_photos',
'https://storage.googleapis.com/download.tensorflow.org/example_images/flower_photos.tgz',
untar=True)
flowers_root = pathlib.Path(flowers_root)
Import swiss_army keras lib
[4]:
#Uncomment to install dependencies, if needed
#!pip3 install --upgrade git+https://github.com/waterviewsrl/swiss-army-keras.git
#!pip3 install typeguard
#!pip3 install --upgrade irondomo
#!pip3 install --upgrade pillow
#!pip3 install --upgrade adabelief_tf
from swiss_army_keras.dataset_utils import ClassificationAlbumentationsDataLoader
from swiss_army_keras import models, base, utils, losses, optimizers
Define input tensor and build classifier model with default MobileNetV3Large minimalistic backbone
This model (distiller) defines a top classification layer which tries to go beyond the usual GlobalPooling + Dense layer, by preserving information about spatial relation of macrofeatures in the last convolutional layer output.
[5]:
inp = tf.keras.layers.Input(shape=(512, 512, 3), batch_size=1)
model = models.distiller_classifier(inp, 5, macrofeatures_number=5, size=256)
WARNING:tensorflow:`input_shape` is undefined or non-square, or `rows` is not 224. Weights for input shape (224, 224) will be loaded as the default.
Define augmentation pipeline (see https://albumentations.ai/docs/)
[6]:
def get_training_augmentation(width=512, height=512):
train_transform = [
#albu.RandomRotate90(p=0.5),
#albu.OneOf([
#
#
#], p=1),
#albu.Resize(width, height, 2, p=1),
#albu.ShiftScaleRotate(shift_limit = 0.05, scale_limit=(0.1, 0.1), interpolation=2, rotate_limit=25, p=0.3),
albu.HorizontalFlip(p=0.5),
#albu.VerticalFlip(p=0.15),
albu.Compose([
albu.OneOf([
albu.ISONoise(p=1),
albu.MultiplicativeNoise(p=1),
albu.GaussNoise(p=1),
albu.ImageCompression(p=1),
],
p=0.2,
),
#albu.Perspective(pad_mode=2, p=0.1),
albu.OneOf(
[
albu.CLAHE(p=1),
albu.RandomBrightnessContrast(p=1),
albu.Sharpen(p=1),
albu.Emboss(p=1),
albu.RandomGamma(p=1),
],
p=0.5,
),
albu.OneOf(
[
albu.Blur(blur_limit=5, p=1),
albu.MedianBlur(blur_limit=5, p=1),
albu.MotionBlur(blur_limit=5, p=1),
],
p=0.25,
),
albu.OneOf(
[
albu.RandomBrightnessContrast(p=1),
albu.HueSaturationValue(p=1),
albu.RGBShift(p=1),
],
p=0.25,
),
albu.OneOf(
[
#albu.ElasticTransform(alpha=10, sigma=10 * 0.05, alpha_affine=10 * 0.03, p=0.5),
albu.GridDistortion(p=0.5),
albu.OpticalDistortion(distort_limit=0.3, shift_limit=0.05, p=1)
],
p=0.25,
)], p=0.5),
#DropsOnLens(maxR=40, minR=30, p=0.1),
albu.ToGray(p=0.2)
]
return albu.Compose(train_transform)
#return albu.Compose([])
Initialize classification dataloader
This will create a number of workers equal to half the number of CPU cores on the machine. These workers will produce random augmentations according to the defined pipeline, so that at each epoch every batch will change.
Please note: Passing normalization=model.preprocessing grants that the correct normalization is applied in a consistent way during training, evaluation and quantization.
Please note: This will produce a lot of output, depending also on the number of CPUs, to monitor workers connectivity and liveliness
[7]:
dl = ClassificationAlbumentationsDataLoader(str(flowers_root), width=512, height=512, train_augmentations=get_training_augmentation(), batch_size=16, normalization=model.preprocessing)
WARNING:root:Normalizing in range: [ 0 255]
Class label daisy will have id: 0
Class label dandelion will have id: 1
Class label roses will have id: 2
Class label sunflowers will have id: 3
Class label tulips will have id: 4
train_val_test_split: [2936, 367, 367]
WARNING:root:KEYS: None
WARNING:root:Router on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:KEYS: None
WARNING:root:KEYS: (b'P+S690P{iVPfx<aFJwxfSY^ugFzjuWOnaIh!o7J<', b':$80ST.hxA5xL7c+@$3YTEohOR^GhrJ2$qzN@bR^')
WARNING:root:KEYS: None
WARNING:root:Attaching HOST CURVE keys.
WARNING:root:Dealer on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 128, 'value': 96, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CLOSED'}
WARNING:root:Router on "ipc:///tmp/swiss_army_keras_classification_curve_socket_QbuLn4Q="
WARNING:root:Dealer on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:KEYS: None
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4, 'value': 297, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECT_RETRIED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 128, 'value': 97, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CLOSED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 8, 'value': 107, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_LISTENING'}
WARNING:root:Dealer on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:KEYS: None
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4, 'value': 297, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECT_RETRIED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_curve_socket_QbuLn4Q=): {'event': 8, 'value': 108, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_curve_socket_QbuLn4Q=', 'description': 'EVENT_LISTENING'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 1, 'value': 98, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 32, 'value': 109, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_ACCEPTED'}
WARNING:root:Dealer on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:KEYS: None
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 1, 'value': 99, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 32, 'value': 115, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_ACCEPTED'}
WARNING:root:Dealer on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:KEYS: None
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 1, 'value': 100, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 32, 'value': 116, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_ACCEPTED'}
WARNING:root:Dealer on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:KEYS: None
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 1, 'value': 101, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 32, 'value': 112, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_ACCEPTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Dealer on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:KEYS: None
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 32, 'value': 121, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_ACCEPTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 1, 'value': 103, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Dealer on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 1, 'value': 96, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECTED'}
WARNING:root:KEYS: None
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 32, 'value': 125, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_ACCEPTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:KEYS: None
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 1, 'value': 104, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 32, 'value': 126, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_ACCEPTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Dealer on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 1, 'value': 97, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECTED'}
WARNING:root:Dealer on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 32, 'value': 127, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_ACCEPTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 1, 'value': 105, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 1, 'value': 106, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECTED'}
WARNING:root:KEYS: None
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 32, 'value': 110, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_ACCEPTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 32, 'value': 111, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_ACCEPTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Dealer on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:KEYS: None
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 1, 'value': 107, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 32, 'value': 129, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_ACCEPTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Dealer on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:KEYS: None
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 1, 'value': 108, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 32, 'value': 131, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_ACCEPTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Dealer on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:KEYS: None
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 1, 'value': 109, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 32, 'value': 130, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_ACCEPTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Dealer on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 1, 'value': 110, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECTED'}
WARNING:root:KEYS: None
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 32, 'value': 120, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_ACCEPTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Dealer on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:KEYS: None
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 32, 'value': 113, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_ACCEPTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 1, 'value': 112, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Dealer on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:KEYS: None
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 32, 'value': 114, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_ACCEPTED'}
WARNING:root:KEYS: None
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 1, 'value': 112, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Dealer on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:Dealer on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:KEYS: None
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 32, 'value': 117, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_ACCEPTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 1, 'value': 112, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 1, 'value': 114, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Dealer on "ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q="
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 32, 'value': 137, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_ACCEPTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 1, 'value': 113, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_CONNECTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 32, 'value': 134, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_ACCEPTED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
WARNING:root:Event Monitor(Host: ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=): {'event': 4096, 'value': 0, 'endpoint': b'ipc:///tmp/swiss_army_keras_classification_clear_socket_QbuLn4Q=', 'description': 'EVENT_HANDSHAKE_SUCCEEDED'}
Define train val and test sets
[8]:
train_set, val_set, test_set = dl.build_datasets()
View a batch of augmentetd images from train set
[9]:
dl.show_images(16)
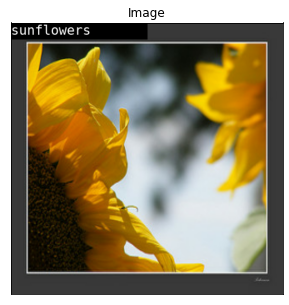
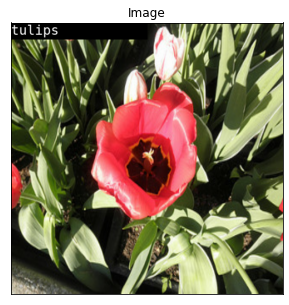
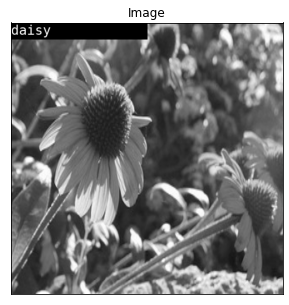
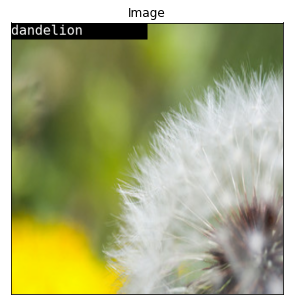
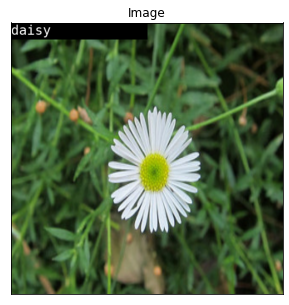
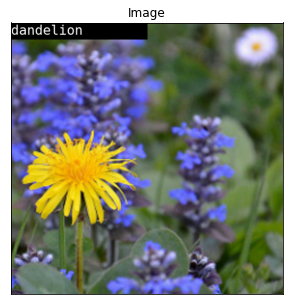
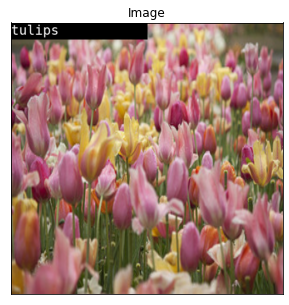
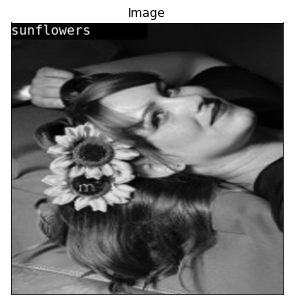
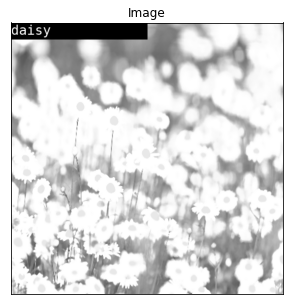
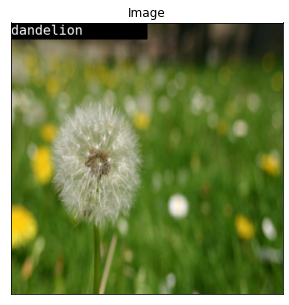
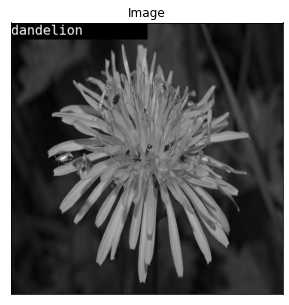
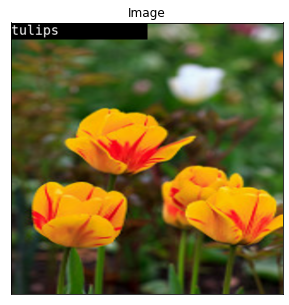
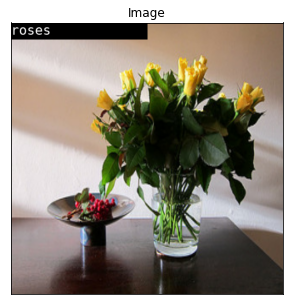
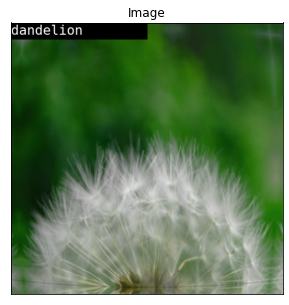
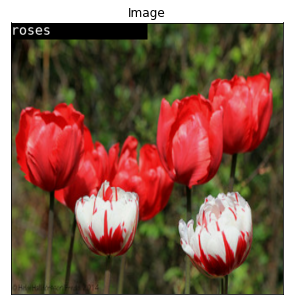
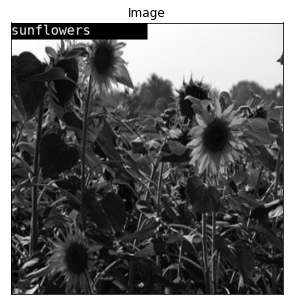
[10]:
from adabelief_tf import AdaBeliefOptimizer
from swiss_army_keras.training_utils import TrainingDriver
[11]:
accuracy = tf.keras.metrics.CategoricalAccuracy()
Train for 40 eochs (20 with frozen backend + 20 with unfrozen backend) with the desired optimizer, loss and metrices.
This will also produce h5 model, f16 and ui8 quantized models, metrics and loss history graphs, all named according to the name (prova_multi in this case) + startup datetime.
Please Note: TrainingDriver implicitly defines callbacks for ModelCheckpoint and for EarlyStopping with 15 epochs patience. The saved models, quantized and not, corresponds to the best validation loss found during training.
[12]:
td = TrainingDriver(model, 'prova_multi', AdaBeliefOptimizer(learning_rate=1e-4, epsilon=1e-14, rectify=True), tf.keras.losses.CategoricalCrossentropy(label_smoothing=0.1), [accuracy], train_set, val_set, test_set, 20 )
td.run()
Please check your arguments if you have upgraded adabelief-tf from version 0.0.1.
Modifications to default arguments:
eps weight_decouple rectify
----------------------- ----- ----------------- -------------
adabelief-tf=0.0.1 1e-08 Not supported Not supported
>=0.1.0 (Current 0.2.1) 1e-14 supported default: True
SGD better than Adam (e.g. CNN for Image Classification) Adam better than SGD (e.g. Transformer, GAN)
---------------------------------------------------------- ----------------------------------------------
Recommended epsilon = 1e-7 Recommended epsilon = 1e-14
For a complete table of recommended hyperparameters, see
https://github.com/juntang-zhuang/Adabelief-Optimizer
You can disable the log message by setting "print_change_log = False", though it is recommended to keep as a reminder.
Model: "model"
__________________________________________________________________________________________________
Layer (type) Output Shape Param # Connected to
==================================================================================================
input_1 (InputLayer) [(1, 512, 512, 3)] 0
__________________________________________________________________________________________________
MobileNetV3Large_backbone (Func [(1, 256, 256, 64), 1437608 input_1[0][0]
__________________________________________________________________________________________________
average_pooling2d (AveragePooli (1, 5, 5, 960) 0 MobileNetV3Large_backbone[0][4]
__________________________________________________________________________________________________
depthwise_conv2d (DepthwiseConv (1, 1, 1, 960) 24960 average_pooling2d[0][0]
__________________________________________________________________________________________________
depthwise_conv2d_1 (DepthwiseCo (1, 1, 1, 960) 24960 average_pooling2d[0][0]
__________________________________________________________________________________________________
depthwise_conv2d_2 (DepthwiseCo (1, 1, 1, 960) 24960 average_pooling2d[0][0]
__________________________________________________________________________________________________
depthwise_conv2d_3 (DepthwiseCo (1, 1, 1, 960) 24960 average_pooling2d[0][0]
__________________________________________________________________________________________________
depthwise_conv2d_4 (DepthwiseCo (1, 1, 1, 960) 24960 average_pooling2d[0][0]
__________________________________________________________________________________________________
flatten (Flatten) (1, 960) 0 depthwise_conv2d[0][0]
__________________________________________________________________________________________________
flatten_1 (Flatten) (1, 960) 0 depthwise_conv2d_1[0][0]
__________________________________________________________________________________________________
flatten_2 (Flatten) (1, 960) 0 depthwise_conv2d_2[0][0]
__________________________________________________________________________________________________
flatten_3 (Flatten) (1, 960) 0 depthwise_conv2d_3[0][0]
__________________________________________________________________________________________________
flatten_4 (Flatten) (1, 960) 0 depthwise_conv2d_4[0][0]
__________________________________________________________________________________________________
dropout (Dropout) (1, 960) 0 flatten[0][0]
__________________________________________________________________________________________________
dropout_1 (Dropout) (1, 960) 0 flatten_1[0][0]
__________________________________________________________________________________________________
dropout_2 (Dropout) (1, 960) 0 flatten_2[0][0]
__________________________________________________________________________________________________
dropout_3 (Dropout) (1, 960) 0 flatten_3[0][0]
__________________________________________________________________________________________________
dropout_4 (Dropout) (1, 960) 0 flatten_4[0][0]
__________________________________________________________________________________________________
concatenate (Concatenate) (1, 4800) 0 dropout[0][0]
dropout_1[0][0]
dropout_2[0][0]
dropout_3[0][0]
dropout_4[0][0]
__________________________________________________________________________________________________
dense (Dense) (1, 256) 1229056 concatenate[0][0]
__________________________________________________________________________________________________
dropout_5 (Dropout) (1, 256) 0 dense[0][0]
__________________________________________________________________________________________________
dense_1 (Dense) (1, 5) 1285 dropout_5[0][0]
==================================================================================================
Total params: 2,792,749
Trainable params: 1,355,141
Non-trainable params: 1,437,608
__________________________________________________________________________________________________
Epoch 1/20
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
/usr/local/lib/python3.6/dist-packages/keras/utils/generic_utils.py:497: CustomMaskWarning: Custom mask layers require a config and must override get_config. When loading, the custom mask layer must be passed to the custom_objects argument.
category=CustomMaskWarning)
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
183/183 [==============================] - ETA: 0s - loss: 2.0143 - categorical_accuracy: 0.5249WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
183/183 [==============================] - 16s 55ms/step - loss: 2.0143 - categorical_accuracy: 0.5249 - val_loss: 1.8842 - val_categorical_accuracy: 0.6591
Epoch 2/20
183/183 [==============================] - 10s 50ms/step - loss: 1.6382 - categorical_accuracy: 0.7510 - val_loss: 1.4155 - val_categorical_accuracy: 0.8040
Epoch 3/20
183/183 [==============================] - 10s 52ms/step - loss: 1.2311 - categorical_accuracy: 0.8463 - val_loss: 1.1303 - val_categorical_accuracy: 0.8438
Epoch 4/20
183/183 [==============================] - 10s 51ms/step - loss: 1.0534 - categorical_accuracy: 0.8736 - val_loss: 1.0248 - val_categorical_accuracy: 0.8551
Epoch 5/20
183/183 [==============================] - 10s 54ms/step - loss: 0.9722 - categorical_accuracy: 0.8863 - val_loss: 0.9528 - val_categorical_accuracy: 0.8693
Epoch 6/20
183/183 [==============================] - 10s 53ms/step - loss: 0.9048 - categorical_accuracy: 0.8975 - val_loss: 0.8957 - val_categorical_accuracy: 0.8807
Epoch 7/20
183/183 [==============================] - 10s 51ms/step - loss: 0.8534 - categorical_accuracy: 0.9088 - val_loss: 0.8701 - val_categorical_accuracy: 0.8778
Epoch 8/20
183/183 [==============================] - 10s 51ms/step - loss: 0.8143 - categorical_accuracy: 0.9208 - val_loss: 0.8423 - val_categorical_accuracy: 0.8778
Epoch 9/20
183/183 [==============================] - 10s 53ms/step - loss: 0.7829 - categorical_accuracy: 0.9255 - val_loss: 0.8147 - val_categorical_accuracy: 0.8864
Epoch 10/20
183/183 [==============================] - 10s 53ms/step - loss: 0.7551 - categorical_accuracy: 0.9324 - val_loss: 0.7910 - val_categorical_accuracy: 0.8977
Epoch 11/20
183/183 [==============================] - 10s 53ms/step - loss: 0.7279 - categorical_accuracy: 0.9372 - val_loss: 0.7781 - val_categorical_accuracy: 0.8977
Epoch 12/20
183/183 [==============================] - 10s 53ms/step - loss: 0.7150 - categorical_accuracy: 0.9430 - val_loss: 0.7659 - val_categorical_accuracy: 0.9006
Epoch 13/20
183/183 [==============================] - 10s 53ms/step - loss: 0.6990 - categorical_accuracy: 0.9406 - val_loss: 0.7563 - val_categorical_accuracy: 0.9006
Epoch 14/20
183/183 [==============================] - 10s 54ms/step - loss: 0.6879 - categorical_accuracy: 0.9423 - val_loss: 0.7415 - val_categorical_accuracy: 0.8977
Epoch 15/20
183/183 [==============================] - 10s 52ms/step - loss: 0.6765 - categorical_accuracy: 0.9491 - val_loss: 0.7342 - val_categorical_accuracy: 0.8977
Epoch 16/20
183/183 [==============================] - 10s 52ms/step - loss: 0.6601 - categorical_accuracy: 0.9529 - val_loss: 0.7322 - val_categorical_accuracy: 0.8977
Epoch 17/20
183/183 [==============================] - 10s 52ms/step - loss: 0.6540 - categorical_accuracy: 0.9532 - val_loss: 0.7276 - val_categorical_accuracy: 0.8920
Epoch 18/20
183/183 [==============================] - 10s 54ms/step - loss: 0.6433 - categorical_accuracy: 0.9583 - val_loss: 0.7173 - val_categorical_accuracy: 0.9091
Epoch 19/20
183/183 [==============================] - 10s 52ms/step - loss: 0.6379 - categorical_accuracy: 0.9577 - val_loss: 0.7139 - val_categorical_accuracy: 0.9034
Epoch 20/20
183/183 [==============================] - 9s 50ms/step - loss: 0.6310 - categorical_accuracy: 0.9604 - val_loss: 0.7095 - val_categorical_accuracy: 0.9062
WARNING:root:Unfreezing Model
Model: "model"
__________________________________________________________________________________________________
Layer (type) Output Shape Param # Connected to
==================================================================================================
input_1 (InputLayer) [(1, 512, 512, 3)] 0
__________________________________________________________________________________________________
MobileNetV3Large_backbone (Func [(1, 256, 256, 64), 1437608 input_1[0][0]
__________________________________________________________________________________________________
average_pooling2d (AveragePooli (1, 5, 5, 960) 0 MobileNetV3Large_backbone[0][4]
__________________________________________________________________________________________________
depthwise_conv2d (DepthwiseConv (1, 1, 1, 960) 24960 average_pooling2d[0][0]
__________________________________________________________________________________________________
depthwise_conv2d_1 (DepthwiseCo (1, 1, 1, 960) 24960 average_pooling2d[0][0]
__________________________________________________________________________________________________
depthwise_conv2d_2 (DepthwiseCo (1, 1, 1, 960) 24960 average_pooling2d[0][0]
__________________________________________________________________________________________________
depthwise_conv2d_3 (DepthwiseCo (1, 1, 1, 960) 24960 average_pooling2d[0][0]
__________________________________________________________________________________________________
depthwise_conv2d_4 (DepthwiseCo (1, 1, 1, 960) 24960 average_pooling2d[0][0]
__________________________________________________________________________________________________
flatten (Flatten) (1, 960) 0 depthwise_conv2d[0][0]
__________________________________________________________________________________________________
flatten_1 (Flatten) (1, 960) 0 depthwise_conv2d_1[0][0]
__________________________________________________________________________________________________
flatten_2 (Flatten) (1, 960) 0 depthwise_conv2d_2[0][0]
__________________________________________________________________________________________________
flatten_3 (Flatten) (1, 960) 0 depthwise_conv2d_3[0][0]
__________________________________________________________________________________________________
flatten_4 (Flatten) (1, 960) 0 depthwise_conv2d_4[0][0]
__________________________________________________________________________________________________
dropout (Dropout) (1, 960) 0 flatten[0][0]
__________________________________________________________________________________________________
dropout_1 (Dropout) (1, 960) 0 flatten_1[0][0]
__________________________________________________________________________________________________
dropout_2 (Dropout) (1, 960) 0 flatten_2[0][0]
__________________________________________________________________________________________________
dropout_3 (Dropout) (1, 960) 0 flatten_3[0][0]
__________________________________________________________________________________________________
dropout_4 (Dropout) (1, 960) 0 flatten_4[0][0]
__________________________________________________________________________________________________
concatenate (Concatenate) (1, 4800) 0 dropout[0][0]
dropout_1[0][0]
dropout_2[0][0]
dropout_3[0][0]
dropout_4[0][0]
__________________________________________________________________________________________________
dense (Dense) (1, 256) 1229056 concatenate[0][0]
__________________________________________________________________________________________________
dropout_5 (Dropout) (1, 256) 0 dense[0][0]
__________________________________________________________________________________________________
dense_1 (Dense) (1, 5) 1285 dropout_5[0][0]
==================================================================================================
Total params: 2,792,749
Trainable params: 2,768,349
Non-trainable params: 24,400
__________________________________________________________________________________________________
Epoch 1/20
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
183/183 [==============================] - ETA: 0s - loss: 0.7263 - categorical_accuracy: 0.9095WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
WARNING:tensorflow:Model was constructed with shape (1, 512, 512, 3) for input KerasTensor(type_spec=TensorSpec(shape=(1, 512, 512, 3), dtype=tf.float32, name='input_1'), name='input_1', description="created by layer 'input_1'"), but it was called on an input with incompatible shape (16, 512, 512, 3).
183/183 [==============================] - 63s 244ms/step - loss: 0.7263 - categorical_accuracy: 0.9095 - val_loss: 0.8143 - val_categorical_accuracy: 0.8608
Epoch 2/20
183/183 [==============================] - 42s 226ms/step - loss: 0.6166 - categorical_accuracy: 0.9611 - val_loss: 0.7439 - val_categorical_accuracy: 0.8807
Epoch 3/20
183/183 [==============================] - 41s 223ms/step - loss: 0.5775 - categorical_accuracy: 0.9775 - val_loss: 0.6824 - val_categorical_accuracy: 0.9062
Epoch 4/20
183/183 [==============================] - 41s 224ms/step - loss: 0.5598 - categorical_accuracy: 0.9843 - val_loss: 0.6625 - val_categorical_accuracy: 0.9091
Epoch 5/20
183/183 [==============================] - 41s 224ms/step - loss: 0.5425 - categorical_accuracy: 0.9870 - val_loss: 0.6520 - val_categorical_accuracy: 0.9006
Epoch 6/20
183/183 [==============================] - 41s 225ms/step - loss: 0.5324 - categorical_accuracy: 0.9908 - val_loss: 0.6757 - val_categorical_accuracy: 0.8892
Epoch 7/20
183/183 [==============================] - 42s 228ms/step - loss: 0.5218 - categorical_accuracy: 0.9911 - val_loss: 0.6407 - val_categorical_accuracy: 0.9176
Epoch 8/20
183/183 [==============================] - 41s 223ms/step - loss: 0.5139 - categorical_accuracy: 0.9928 - val_loss: 0.6174 - val_categorical_accuracy: 0.9318
Epoch 9/20
183/183 [==============================] - 42s 225ms/step - loss: 0.5096 - categorical_accuracy: 0.9915 - val_loss: 0.6094 - val_categorical_accuracy: 0.9261
Epoch 10/20
183/183 [==============================] - 42s 227ms/step - loss: 0.4980 - categorical_accuracy: 0.9949 - val_loss: 0.6024 - val_categorical_accuracy: 0.9318
Epoch 11/20
183/183 [==============================] - 42s 228ms/step - loss: 0.4962 - categorical_accuracy: 0.9928 - val_loss: 0.6056 - val_categorical_accuracy: 0.9290
Epoch 12/20
183/183 [==============================] - 42s 226ms/step - loss: 0.4871 - categorical_accuracy: 0.9956 - val_loss: 0.6182 - val_categorical_accuracy: 0.9318
Epoch 13/20
183/183 [==============================] - 42s 225ms/step - loss: 0.4838 - categorical_accuracy: 0.9949 - val_loss: 0.5962 - val_categorical_accuracy: 0.9318
Epoch 14/20
183/183 [==============================] - 41s 223ms/step - loss: 0.4812 - categorical_accuracy: 0.9945 - val_loss: 0.5968 - val_categorical_accuracy: 0.9375
Epoch 15/20
183/183 [==============================] - 41s 222ms/step - loss: 0.4762 - categorical_accuracy: 0.9959 - val_loss: 0.5996 - val_categorical_accuracy: 0.9290
Epoch 16/20
183/183 [==============================] - 41s 224ms/step - loss: 0.4726 - categorical_accuracy: 0.9956 - val_loss: 0.5892 - val_categorical_accuracy: 0.9347
Epoch 17/20
183/183 [==============================] - 41s 224ms/step - loss: 0.4675 - categorical_accuracy: 0.9973 - val_loss: 0.5873 - val_categorical_accuracy: 0.9318
Epoch 18/20
183/183 [==============================] - 41s 224ms/step - loss: 0.4639 - categorical_accuracy: 0.9966 - val_loss: 0.5792 - val_categorical_accuracy: 0.9318
Epoch 19/20
183/183 [==============================] - 42s 225ms/step - loss: 0.4599 - categorical_accuracy: 0.9980 - val_loss: 0.5845 - val_categorical_accuracy: 0.9318
Epoch 20/20
183/183 [==============================] - 41s 222ms/step - loss: 0.4561 - categorical_accuracy: 0.9973 - val_loss: 0.5657 - val_categorical_accuracy: 0.9403
WARNING:root:Quantizing Model
WARNING:tensorflow:Compiled the loaded model, but the compiled metrics have yet to be built. `model.compile_metrics` will be empty until you train or evaluate the model.
WARNING:tensorflow:Compiled the loaded model, but the compiled metrics have yet to be built. `model.compile_metrics` will be empty until you train or evaluate the model.
INFO:tensorflow:Assets written to: /tmp/tmp2chah2d2/assets
INFO:tensorflow:Assets written to: /tmp/tmp2chah2d2/assets
/usr/local/lib/python3.6/dist-packages/keras/utils/generic_utils.py:497: CustomMaskWarning: Custom mask layers require a config and must override get_config. When loading, the custom mask layer must be passed to the custom_objects argument.
category=CustomMaskWarning)
WARNING:tensorflow:Issue encountered when serializing table_initializer.
Type is unsupported, or the types of the items don't match field type in CollectionDef. Note this is a warning and probably safe to ignore.
'NoneType' object has no attribute 'name'
WARNING:tensorflow:Issue encountered when serializing table_initializer.
Type is unsupported, or the types of the items don't match field type in CollectionDef. Note this is a warning and probably safe to ignore.
'NoneType' object has no attribute 'name'
WARNING:absl:For model inputs containing unsupported operations which cannot be quantized, the `inference_input_type` attribute will default to the original type.
WARNING:tensorflow:Compiled the loaded model, but the compiled metrics have yet to be built. `model.compile_metrics` will be empty until you train or evaluate the model.
WARNING:tensorflow:Compiled the loaded model, but the compiled metrics have yet to be built. `model.compile_metrics` will be empty until you train or evaluate the model.
INFO:tensorflow:Assets written to: /tmp/tmpjiq88zc4/assets
INFO:tensorflow:Assets written to: /tmp/tmpjiq88zc4/assets
/usr/local/lib/python3.6/dist-packages/keras/utils/generic_utils.py:497: CustomMaskWarning: Custom mask layers require a config and must override get_config. When loading, the custom mask layer must be passed to the custom_objects argument.
category=CustomMaskWarning)
WARNING:tensorflow:Issue encountered when serializing table_initializer.
Type is unsupported, or the types of the items don't match field type in CollectionDef. Note this is a warning and probably safe to ignore.
'NoneType' object has no attribute 'name'
WARNING:tensorflow:Issue encountered when serializing table_initializer.
Type is unsupported, or the types of the items don't match field type in CollectionDef. Note this is a warning and probably safe to ignore.
'NoneType' object has no attribute 'name'
<Figure size 432x288 with 0 Axes>
Show some reuslts with corresponding reference classification
[13]:
dl.show_results(model, 16)
<Figure size 1584x1584 with 0 Axes>
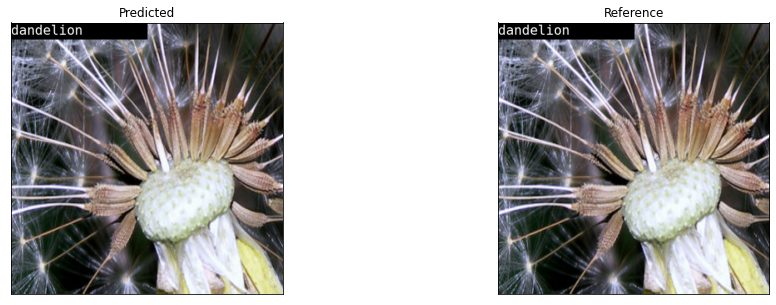
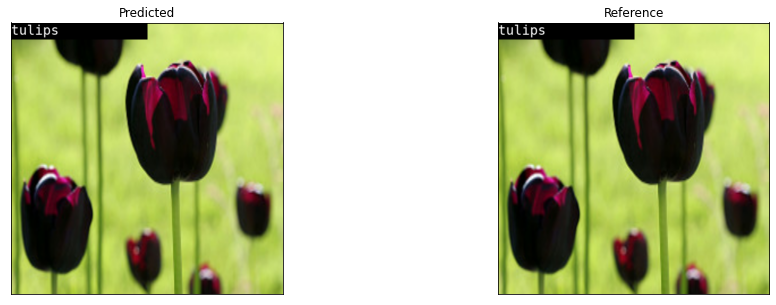
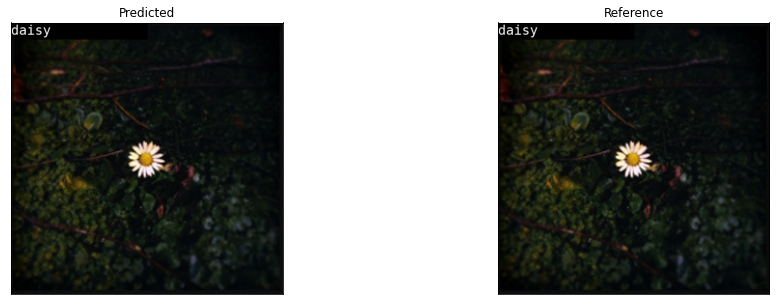
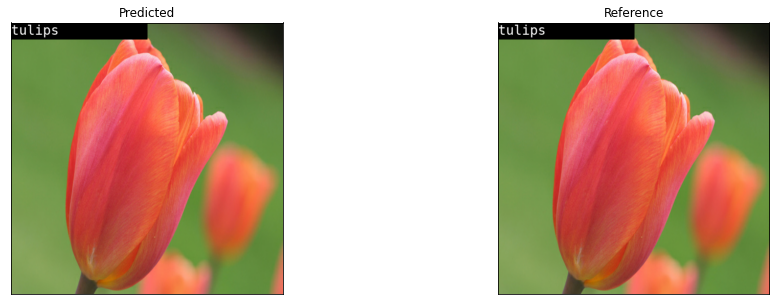
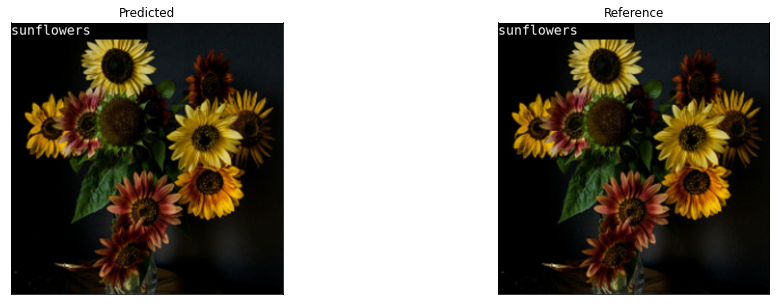
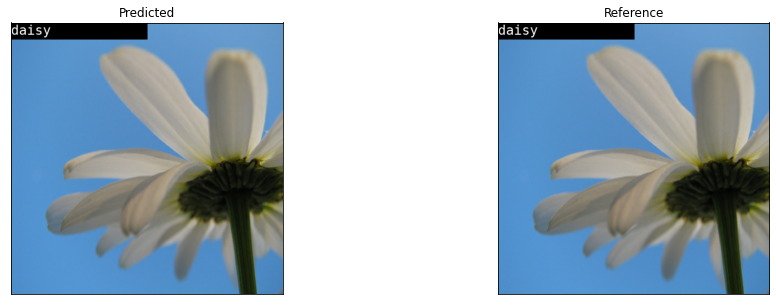
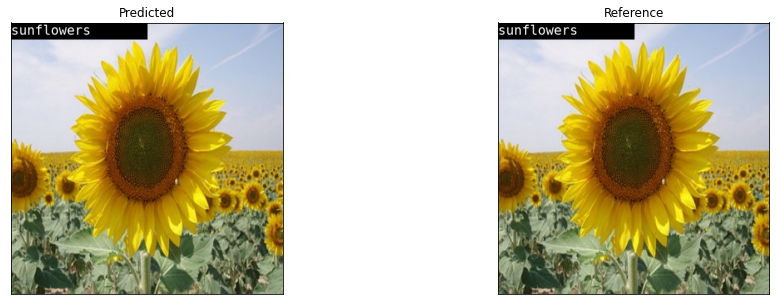
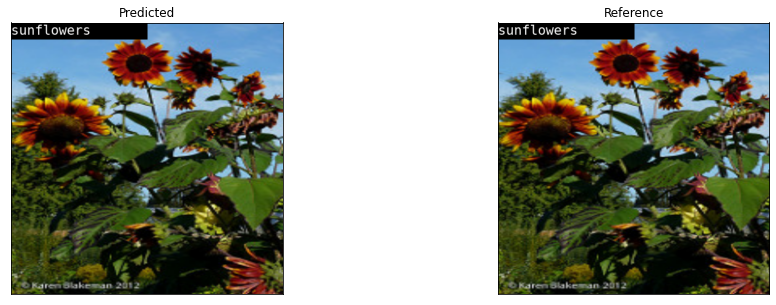
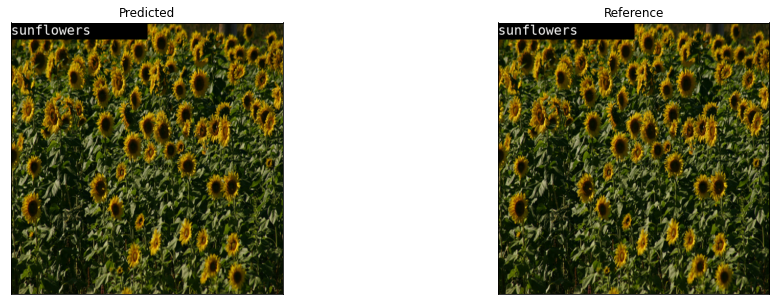
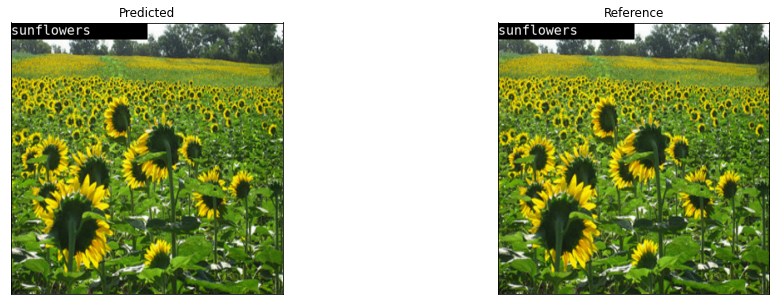
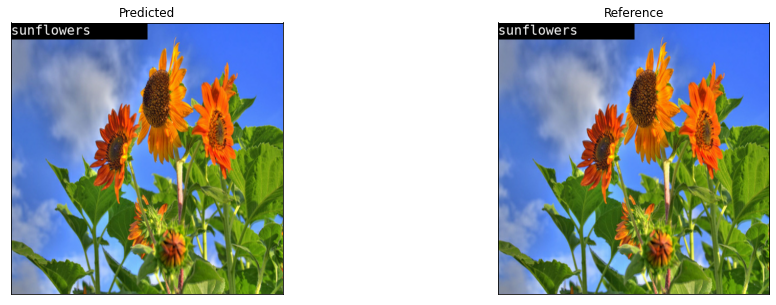
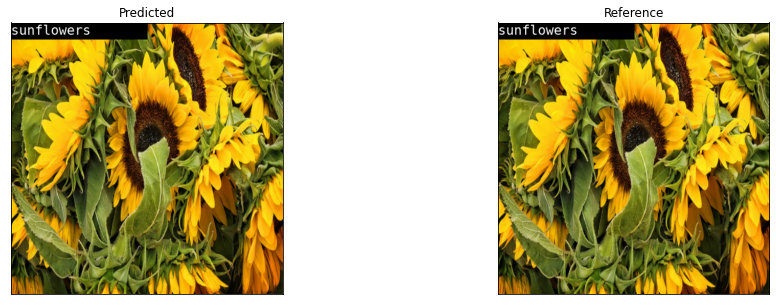
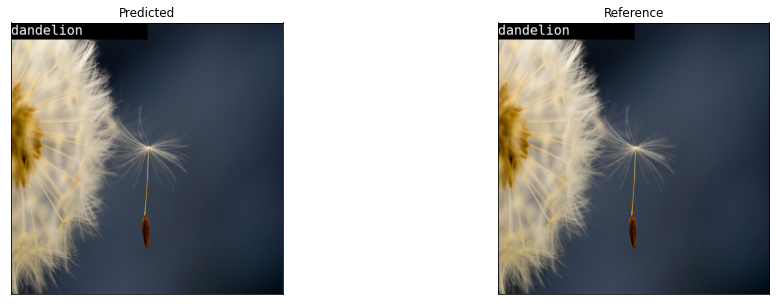
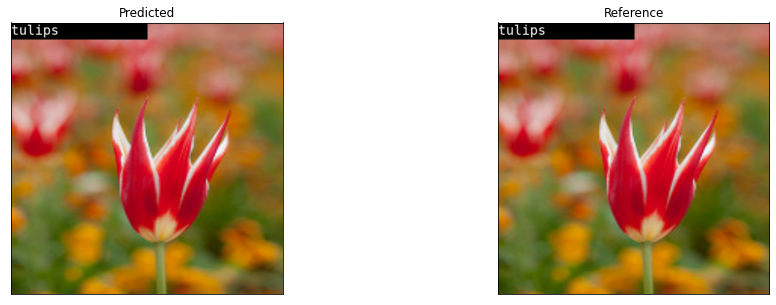
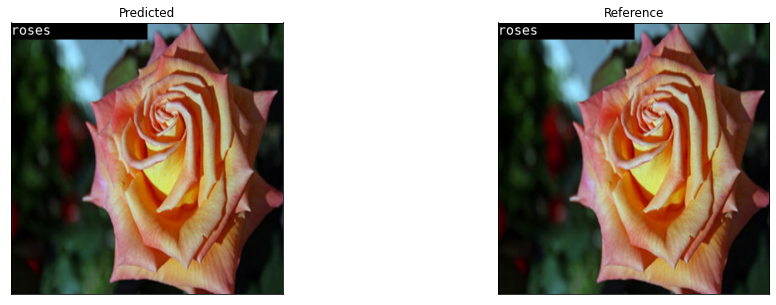
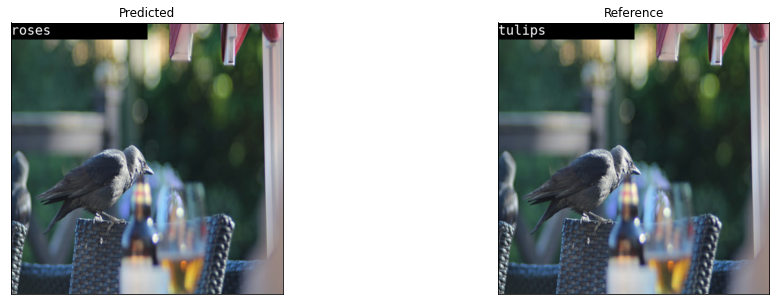
[ ]:
[ ]:
[ ]:
[ ]: